React Overview
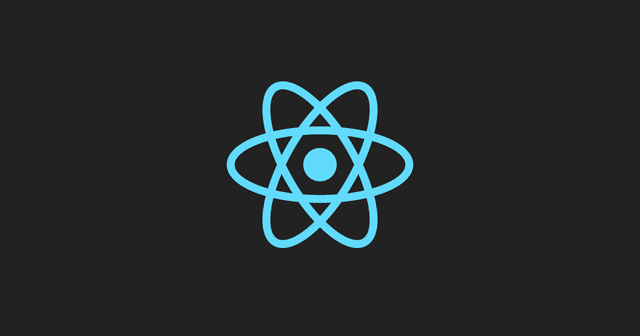
What is React?
- A JS library for building User Interfaces
- A Single Page Application that utilizes the virtual DOM to greatly improve performance as the whole DOM is already downlaoded annd just what needs to be displayed is rendered.
What are components in React?
- Components make up the visual layer of our applicaiton and lets us create re-usable but seperate pieces of code
- Components are class or functions that return JSX
- Components can be nested as deep as you want
What are Hooks?
- Hooks are features within React that let you use functional components to interact with the state, lifecycle and more.
- Hooks changed teh way most developers use React these days and class components aren't seen as much anymore.
- Hookes are essential, and their name derives from their ability to "Hook the state"
- Hooks include:
- useState
- useEffect
- useContext
- useReducer
- useCallback
- useMemo
- useRef
- many more...
- Hooks include:
What is JSX?
- JSX is a hybrid of JavaScript and HTML code that React can read.
- Browsers don't know how to read JSX natively so the code will need to be compiled.
What is React-Router?
- React router lets us have multiple pages in our React
- Using BrowserRouter, Router, Route, Routes to achieve this.
What are Props?
- Props are how we pass data down from one parent component to a child
- Props are short for properties and can be passed down to multiple children
- Use the "this" keyword when interacting with props from a class based component
- Prop Drilling is the act of passing props down mulitple components.
props.js
1import React from 'react'; 2import ReactDOM from 'react-dom/client'; 3 4function Car(props) { 5 return <h2>I am a { props.brand }!</h2>; 6} 7 8function Garage() { 9 return ( 10 <> 11 <h1>Who lives in my garage?</h1> 12 <Car brand="Ford" /> 13 </> 14 ); 15} 16 17const root = ReactDOM.createRoot(document.getElementById('root')); 18root.render(<Garage />); 19
What is State?
- State is a JS object that lets us store data about a particular component
- We don't interact with the state directly and we don't create new variables in place of the state
- setState is how we set the state in class components or functional components with the hooks.
- Lifting the state is used to take data about a particular component and pass it back to a parent that may need that data
state.js
1import React, { useState } from 'react'; 2 3function Example() { 4 // Declare a new state variable, which we'll call "count" 5 const [count, setCount] = useState(0); 6 7 return ( 8 <div> 9 <p>You clicked {count} times</p> 10 <button onClick={() => setCount(count + 1)}> 11 Click me 12 </button> 13 </div> 14 ); 15} 16
What is the component Lifecycle?
-
Initialization
-
Mounting
-
Updating
-
Unmounting
-
Class based components use lifecycle methods suck as componentDidUpdate, componenentDidMount, etc
-
Function based components use the useEffect hook
- If the component is in the original useEffect call it will load on component mount while if you put the component inside our useEffect dependency array it will update everytime the state changes.
-
No dependency passed
1useEffect(() => { 2//Runs on every render 3}); 4
- An Empty Array
1useEffect(() => { 2//Runs only on the first render 3}, []); 4
- Props or state values
1useEffect(() => { 2//Runs on the first render 3//And any time any dependency value changes 4}, [prop, state]); 5
Run only on initial render
1import { useState, useEffect } from "react"; 2import ReactDOM from "react-dom/client"; 3 4function Timer() { 5 const [count, setCount] = useState(0); 6 7 useEffect(() => { 8 setTimeout(() => { 9 setCount((count) => count + 1); 10 }, 1000); 11 }, []); // <- add empty brackets here 12 13 return <h1>I've rendered {count} times!</h1>; 14} 15 16const root = ReactDOM.createRoot(document.getElementById('root')); 17root.render(<Timer />); 18
Run only on variable update
1import { useState, useEffect } from "react"; 2import ReactDOM from "react-dom/client"; 3 4function Counter() { 5 const [count, setCount] = useState(0); 6 const [calculation, setCalculation] = useState(0); 7 8 useEffect(() => { 9 setCalculation(() => count * 2); 10 }, [count]); // <- add the count variable here 11 12 return ( 13 <> 14 <p>Count: {count}</p> 15 <button onClick={() => setCount((c) => c + 1)}>+</button> 16 <p>Calculation: {calculation}</p> 17 </> 18 ); 19} 20 21const root = ReactDOM.createRoot(document.getElementById('root')); 22root.render(<Counter />); 23
What is React Global State Management?
- Sometimes React needs a global state object to keep the app working
- think of a logged in user profile
- Prop drilling isn't practical once our application reaches a certain size
- We can use the ContextAPI or Redux to solve this
When to use Context?
-
We designed the application in which used color props in the ParentClass and passed that props to all component (top to down) without checking that it is required by the child class or not and another issue also here that in case if GrandChildClass component was more deeply nested than it was very difficult to manage. This is the problem that Context solves. Context is designed the share values that can be considered "global" such as preferred language, current user, or theme.
-
These types of data include:
-
Theme data (like dark or light mode)
-
User data (the currently authenticated user)
-
Location-specific data (like user language or locale)
When to use context
1 2const App = () => { 3 return( 4 <ParentClass color= "blue"/> 5 ); 6} 7 8const ParentClass = (props) => ( 9 <ChildClass color= {props.color} /> 10) 11 12const ChildClass = (props) => ( 13 <GrandChildClass color= {props.color} /> 14) 15 16const GrandChildClass = (props) => ( 17 <p>Color: {props.color}</p> 18) 19
4 Steps to using React Context
- Create context using the createContext method.
- Take your created context and wrap the context provider around your component tree.
- Put any value you like on your context provider using the value prop.
- Read that value within any component by using the context consumer.
There are two main components that make up Context
- Provider of Context
- Consumer of Context
-
What is the Virtual DOM
- This allows React to update only specific components in the tree without updating the whole DOM
- Keys will need to be generated for each list item for the VDOM errors to go away
Event Listeners
- camelCase the eventName
- Pass the function we want to execute
Conditional Rendering
1isAuthenticated === true
1 {isAuthenticated && operator}
- Using an inline if/else also known as ternirary is godo for additional logic
Noteable Terminal Commands
-
1npx create-react-app appname
-
1npm start
-
1npm build
React here
Check out this Comprehensive CheatSheet devhints