Material-UI Styled Components Implementation with JSS
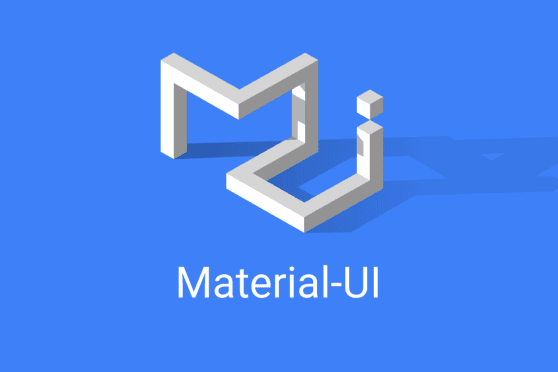
What is Material-UI?
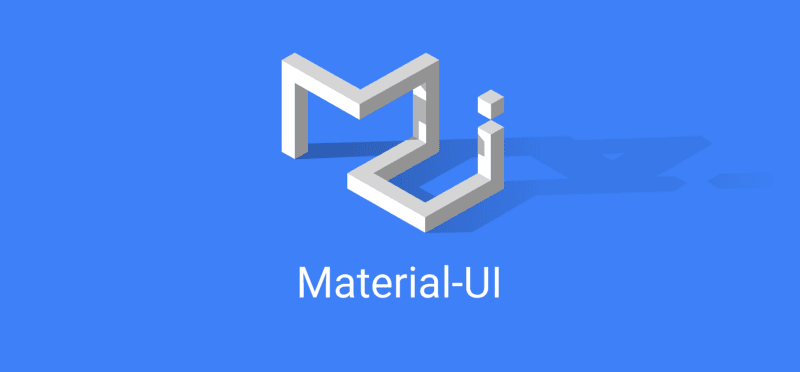
Material-UI | React components for faster and easier web development. Build your own design system, or start with Material Design.
Documentation.
Sometimes we want to to make our own unique styles, Material-UI styled components is a popular method to create your own components styles.
Material-UI lets you create styled compononents with their makeStyle() method.
- Import makeStyles
- Create useStyles object before main function
- Inside our function we can bind useStyles to a variable
- Then we can tap into that variable like the example below with
1className={classes.title}
NPM Installation
1npm install @material-ui/core
1import {makesStyles} from '@material-ui/core' 2 3const useStyles = makeStyles(() => ({ 4 title: { 5 flex: 1, 6 color: 'gold', 7 fontFamily: 'Montserrat', 8 fontWeight: 'bold', 9 cursor: 'pointer', 10 }, 11})); 12 13const Header = () => { 14 const classes = useStyles(); 15 const navigate = useNavigate(); 16 17 const { currency, setCurrency } = CryptoState(); 18 19 const darkTheme = createTheme({ 20 palette: { 21 primary: { 22 main: '#fff', 23 }, 24 type: 'dark', 25 }, 26 }); 27 return ( 28 <ThemeProvider theme={darkTheme}> 29 <AppBar color="transparent" position="static"> 30 <Container> 31 <Toolbar> 32 <Typography 33 variant="h6" 34 onClick={() => { 35 navigate('/'); 36 }} 37 className={classes.title} 38 > 39 Crypto Hunter 40 </Typography> 41 {/* <Button color="inherit">Login</Button> */} 42 <Select 43 variant="outlined" 44 labelId="demo-simple-select-label" 45 id="demo-simple-select" 46 value={currency} 47 style={{ width: 100, height: 40, marginLeft: 15 }} 48 onChange={(e) => setCurrency(e.target.value)} 49 > 50 <MenuItem value={'USD'}>USD</MenuItem> 51 <MenuItem value={'INR'}>INR</MenuItem> 52 </Select> 53 </Toolbar> 54 </Container> 55 </AppBar> 56 </ThemeProvider> 57 ); 58}; 59 60export default Header; 61
Noteables
- This is a a VERY basic implementation.
- There are other ways to implement or pass props, please refer to documentation below
Learn more about it here