Helper Files or Utils
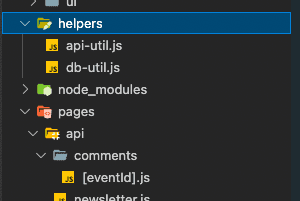
What is Helper file?
One of the well known conventions of React components is that any logic should be broken out of the render function into a helper function. This keeps the component clean, and separates the concerns inside of the component.
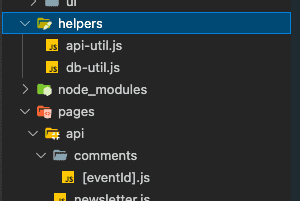
Documentation.
- Referenced Documentation here
Usage
This file contains all utility exposed functions and directly available into other classes and components. Here we need to expose every function which we want to use outside while in the Helper Class file we don’t expose methods directly, we expose only class.
We can create multiple util files as well as per our code structure.
Basic Util
1export function addTwoNumber(number1, number2) { 2 return number1 + number2; 3} 4export function getUserName() { 5 return 'JS Mount'; 6} 7export function getNameArray() { 8 const data = []; 9 for (let i = 0; i < 50; i++) { 10 data.push('JS-' + i); 11 } 12 return data; 13} 14
Usage of Basic Util
1import React from 'react'; 2import { addTwoNumber, getUserName, getNameArray } from './../../util/util'; 3const ComponentA = (props) => { 4 return ( 5 <> 6 <h3>Welcome to Router Component A</h3> 7 <h6>Called util function: Username is {getUserName()}</h6> 8 <h6>Sum of two number (3,4) using util.js - {addTwoNumber(3, 4)}</h6> 9 Total Names: {getNameArray().length} 10 <ul> 11 {getNameArray().map((name) => { 12 return <li>{name}</li>; 13 })} 14 </ul> 15 </> 16 ); 17}; 18export default ComponentA; 19
More Helper Patterns
DB-util
1import { MongoClient } from 'mongodb'; 2 3const dbusername = process.env.mongodb_username; 4const dbpassword = process.env.mongodb_password; 5const dbconnection = '@cluster0.ewevp.mongodb.net/'; 6const dbname = 'nextEvents'; 7const dbprefix = 'mongodb+srv://'; 8 9export async function connectToDatabase() { 10 const client = await MongoClient.connect( 11 `${dbprefix}${dbusername}:${dbpassword}${dbconnection}${dbname}` 12 ); 13 14 return client; 15} 16 17export async function insertDocument(client, collection, document) { 18 const db = client.db(); 19 20 const result = await db.collection(collection).insertOne(document); 21 22 return result; 23} 24 25export async function getAllDocuments(client, collection, sort, filter) { 26 const db = client.db(); 27 28 const documents = await db 29 .collection(collection) 30 .find(filter) 31 .sort(sort) 32 .toArray(); 33 34 return documents; 35} 36
Usage of DB-util
1import { 2 connectToDatabase, 3 insertDocument, 4 getAllDocuments, 5} from '../../../helpers/db-util'; 6 7async function handler(req, res) { 8 const eventId = req.query.eventId; 9 10 let client; 11 12 try { 13 client = await connectToDatabase(); 14 } catch (error) { 15 res.status(500).json({ message: 'Connecting to the database failed!' }); 16 return; 17 } 18 19 if (req.method === 'POST') { 20 const { email, name, text } = req.body; 21 22 if ( 23 !email.includes('@') || 24 !name || 25 name.trim() === '' || 26 !text || 27 text.trim() === '' 28 ) { 29 res.status(422).json({ message: 'Invalid input.' }); 30 client.close(); 31 return; 32 } 33 34 const newComment = { 35 email, 36 name, 37 text, 38 eventId, 39 }; 40 41 let result; 42 43 try { 44 result = await insertDocument(client, 'comments', newComment); 45 newComment._id = result.insertedId; 46 res.status(201).json({ message: 'Added comment.', comment: newComment }); 47 } catch (error) { 48 res.status(500).json({ message: 'Inserting comment failed!' }); 49 } 50 } 51 52 if (req.method === 'GET') { 53 try { 54 const documents = await getAllDocuments( 55 client, 56 'comments', 57 { _id: -1 }, 58 { eventId: eventId } 59 ); 60 res.status(200).json({ comments: documents }); 61 } catch (error) { 62 res.status(500).json({ message: 'Getting comments failed.' }); 63 } 64 } 65 66 client.close(); 67} 68 69export default handler; 70
API-util
1export async function getAllEvents() { 2 const response = await fetch( 3 'https://next-js-course-9dc05-default-rtdb.firebaseio.com/events.json' 4 ); 5 const data = await response.json(); 6 7 const events = []; 8 9 for (const key in data) { 10 events.push({ 11 id: key, 12 ...data[key], 13 }); 14 } 15 16 return events; 17} 18 19export async function getFeaturedEvents() { 20 const allEvents = await getAllEvents(); 21 return allEvents.filter((event) => event.isFeatured); 22} 23 24export async function getEventById(id) { 25 const allEvents = await getAllEvents(); 26 return allEvents.find((event) => event.id === id); 27} 28 29export async function getFilteredEvents(dateFilter) { 30 const { year, month } = dateFilter; 31 32 const allEvents = await getAllEvents(); 33 34 let filteredEvents = allEvents.filter((event) => { 35 const eventDate = new Date(event.date); 36 return ( 37 eventDate.getFullYear() === year && eventDate.getMonth() === month - 1 38 ); 39 }); 40 41 return filteredEvents; 42} 43
Usage of API-util
1import { Fragment } from 'react'; 2import { useRouter } from 'next/router'; 3import Head from 'next/head'; 4 5import { getAllEvents } from '../../helpers/api-util'; 6import EventList from '../../components/events/event-list'; 7import EventsSearch from '../../components/events/events-search'; 8 9function AllEventsPage(props) { 10 const router = useRouter(); 11 const { events } = props; 12 13 function findEventsHandler(year, month) { 14 const fullPath = `/events/${year}/${month}`; 15 16 router.push(fullPath); 17 } 18 19 return ( 20 <Fragment> 21 <Head> 22 <title>All Events</title> 23 <meta 24 name="description" 25 content="Find a lot of great events that allow you to evolve..." 26 /> 27 </Head> 28 <EventsSearch onSearch={findEventsHandler} /> 29 <EventList items={events} /> 30 </Fragment> 31 ); 32} 33 34export async function getStaticProps() { 35 const events = await getAllEvents(); 36 37 return { 38 props: { 39 events: events, 40 }, 41 revalidate: 60, 42 }; 43} 44 45export default AllEventsPage; 46
Noteables
- Remember to create UTIL files early as it can save you time and headache!
Learn more about it here