NextJS: Core Feature Overview
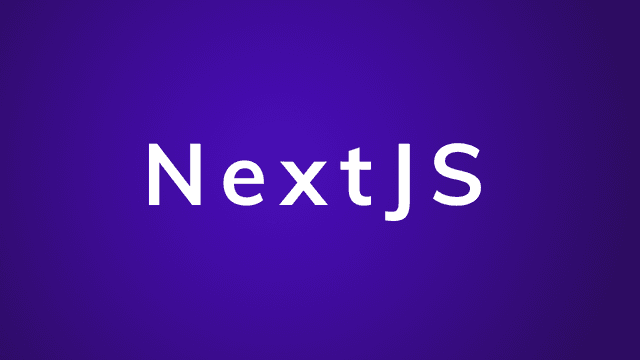
NextJS is a framework for ReactJS.
👀 Wait a second ... a "framework" for React? Isn't React itself already a framework for JavaScript?
Well ... first of all, React is a "library" for JavaScript. That seems to be important for some people.
Not for me, but still, there is a valid point: React already is a framework / library for JavaScript. So it's already an extra layer on top of JS.
Why would we then need NextJS?
Because NextJS makes building React apps easier - especially React apps that should have server-side rendering (though it does way more than just take care of that).
In this article, we'll dive into 3 core concepts and features NextJS has to offer:
- File-based Routing
- Built-in Page Pre-rendering
- Rich Data Fetching Capabilities
File-based Routing
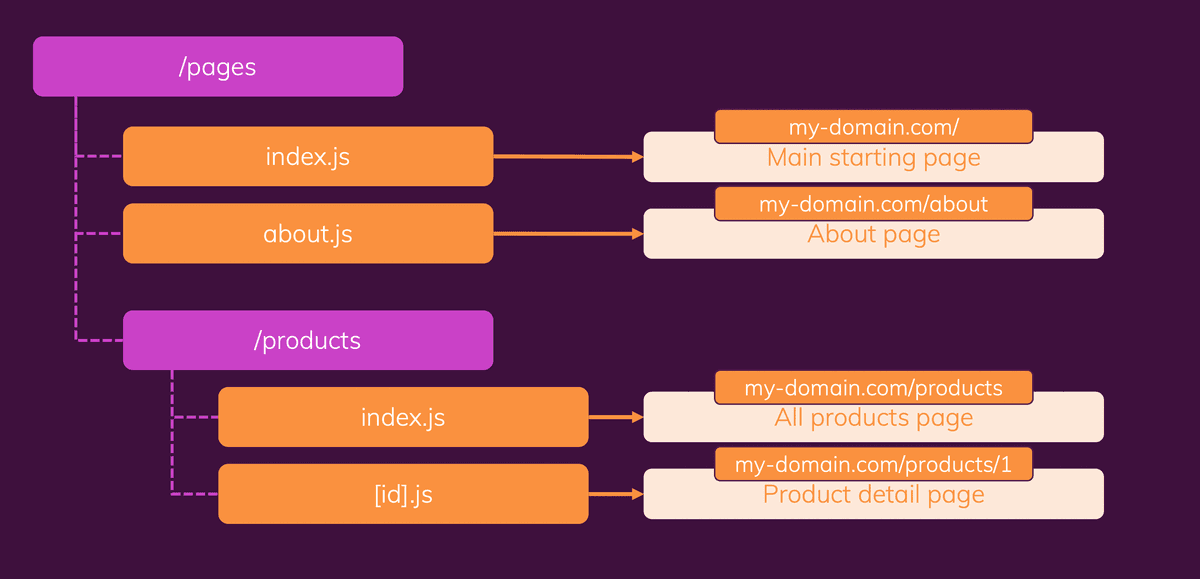
Classic React Rendering
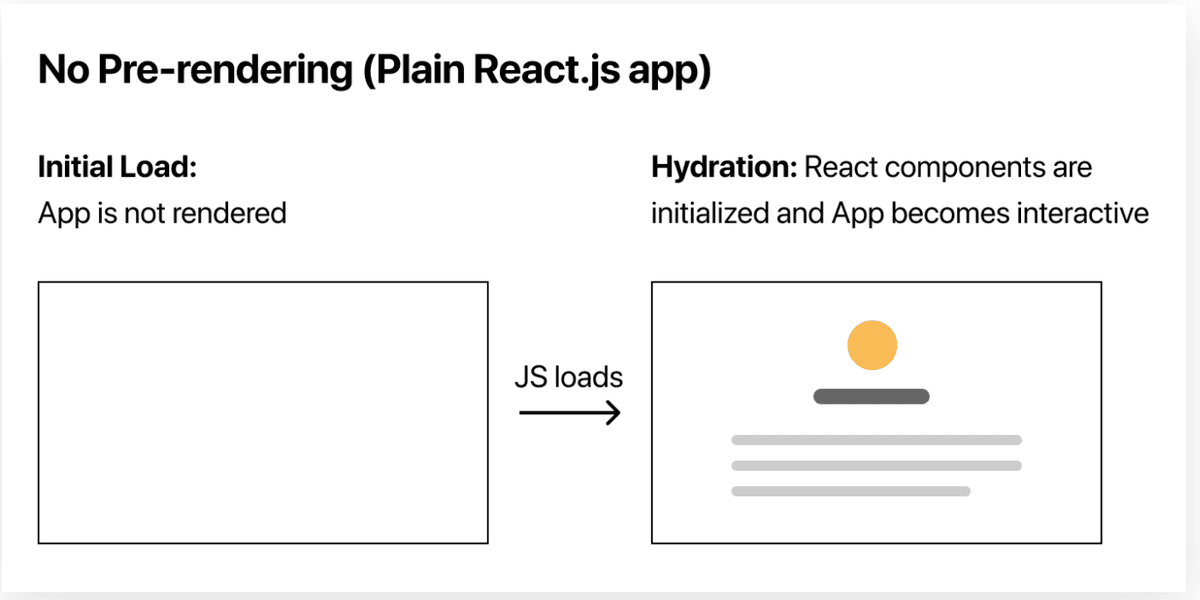
Built-in Page Pre-rendering
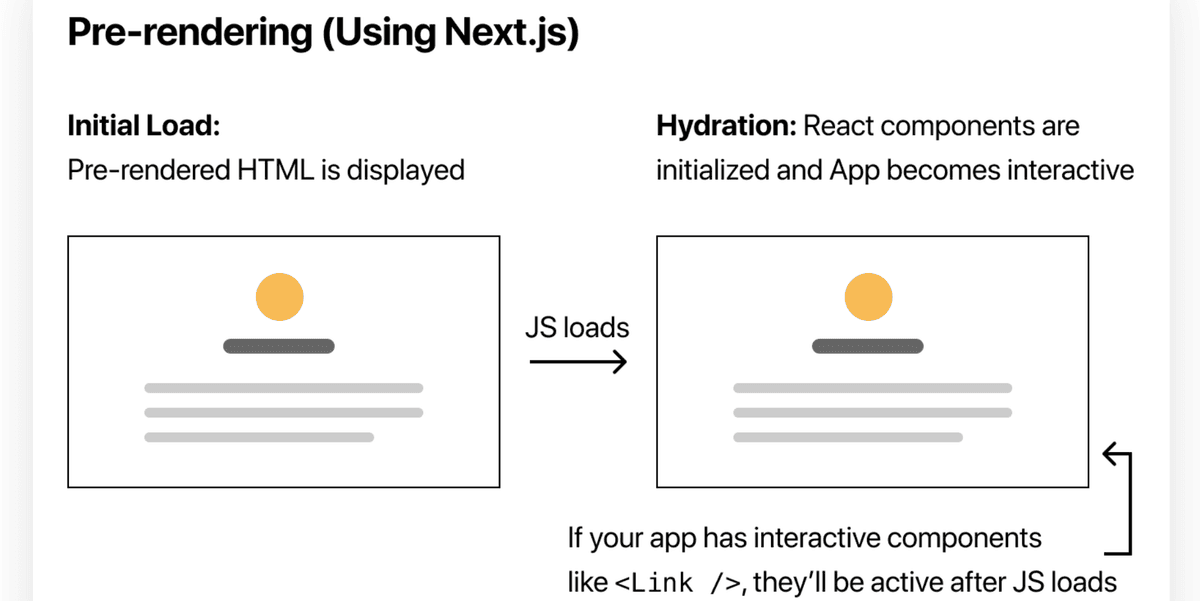
By default, Next.js pre-renders every page. This means that Next.js generates HTML for each page in advance, instead of having it all done by client-side JavaScript. Pre-rendering can result in better performance and SEO. Each generated HTML is associated with minimal JavaScript code necessary for that page. When a page is loaded by the browser, its JavaScript code runs and makes the page fully interactive. (This process is called hydration.)
Two Types of Pre-rendering
Server-Side Rendering
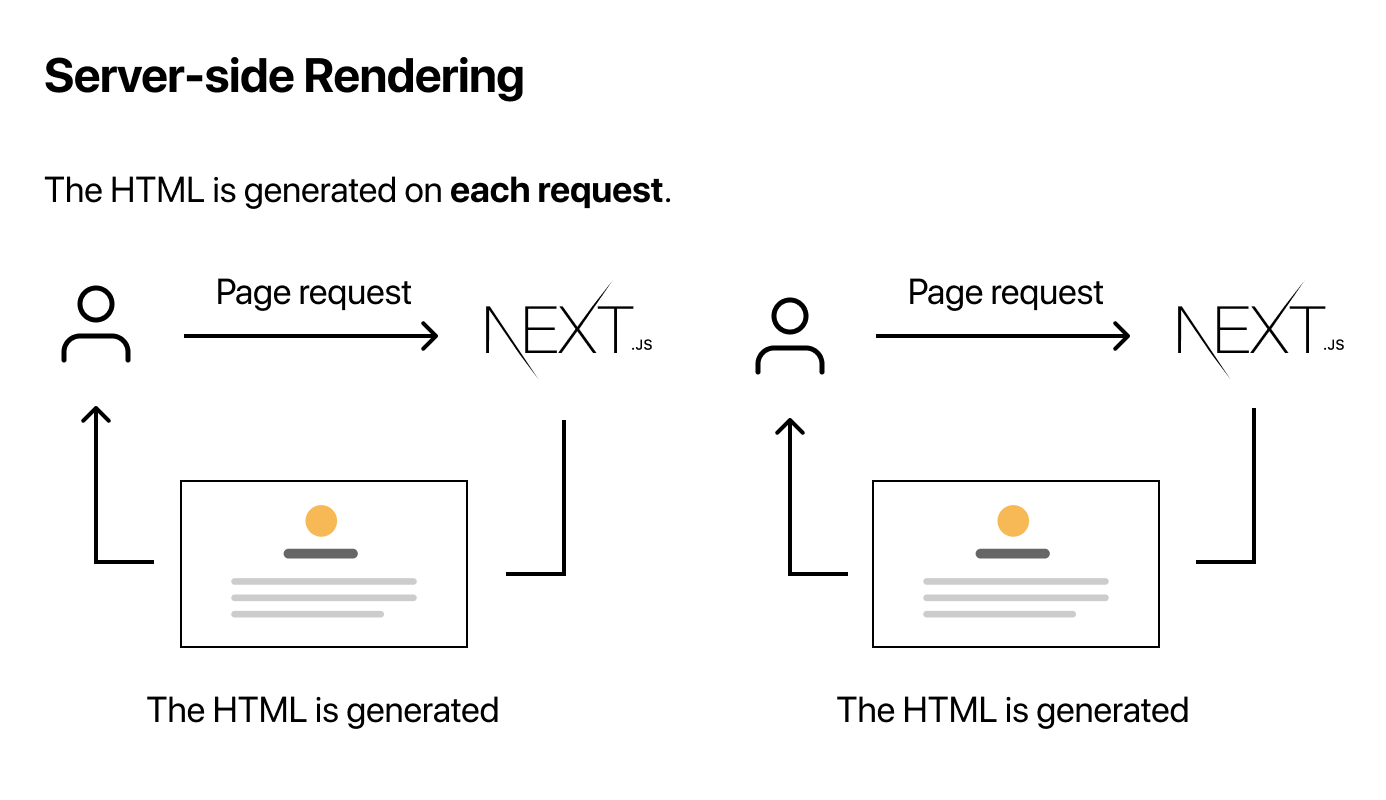
Static Site Generation
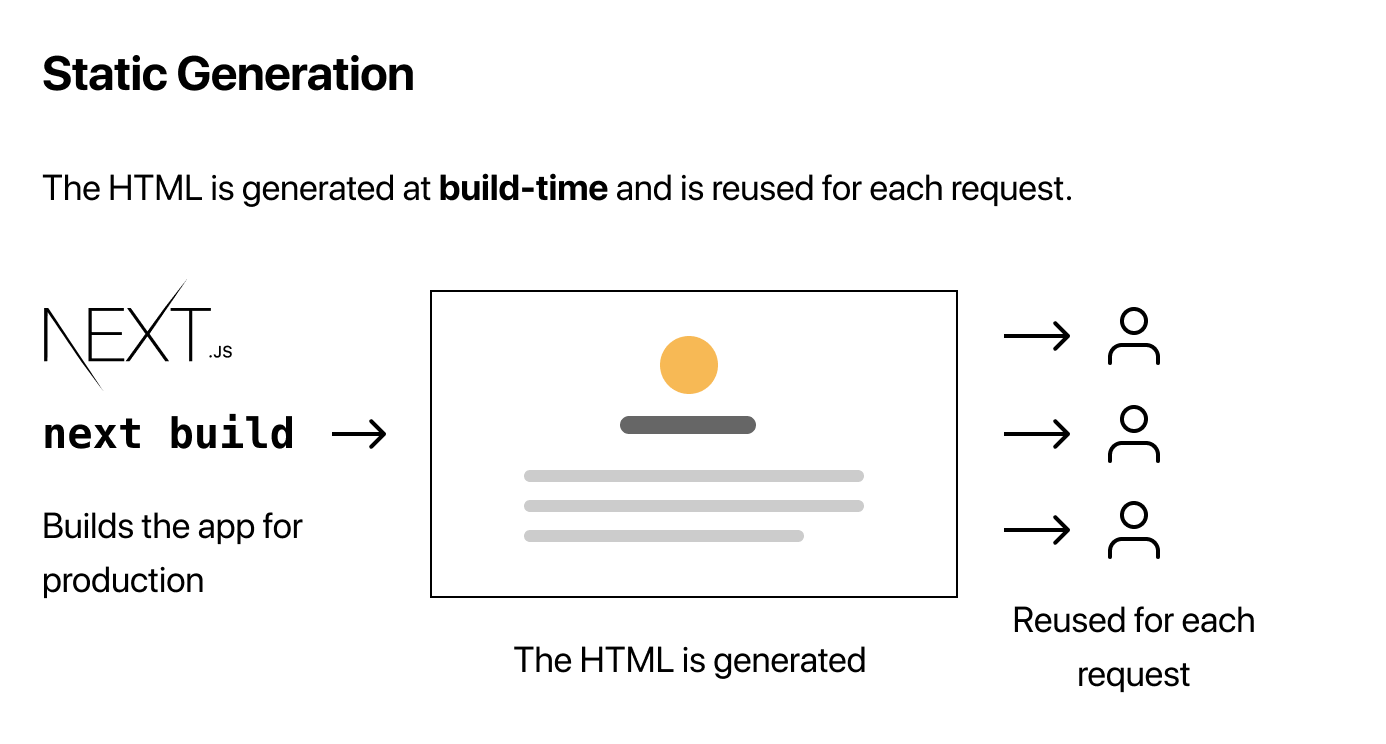
- Next.js has two forms of pre-rendering: Static Generation and Server-side Rendering. The difference is in when it generates the HTML for a page.
- Static Generation is the pre-rendering method that generates the HTML at build time. The pre-rendered HTML is then reused on each request.
- Server-side Rendering is the pre-rendering method that generates the HTML on each request.
Per Page Basis
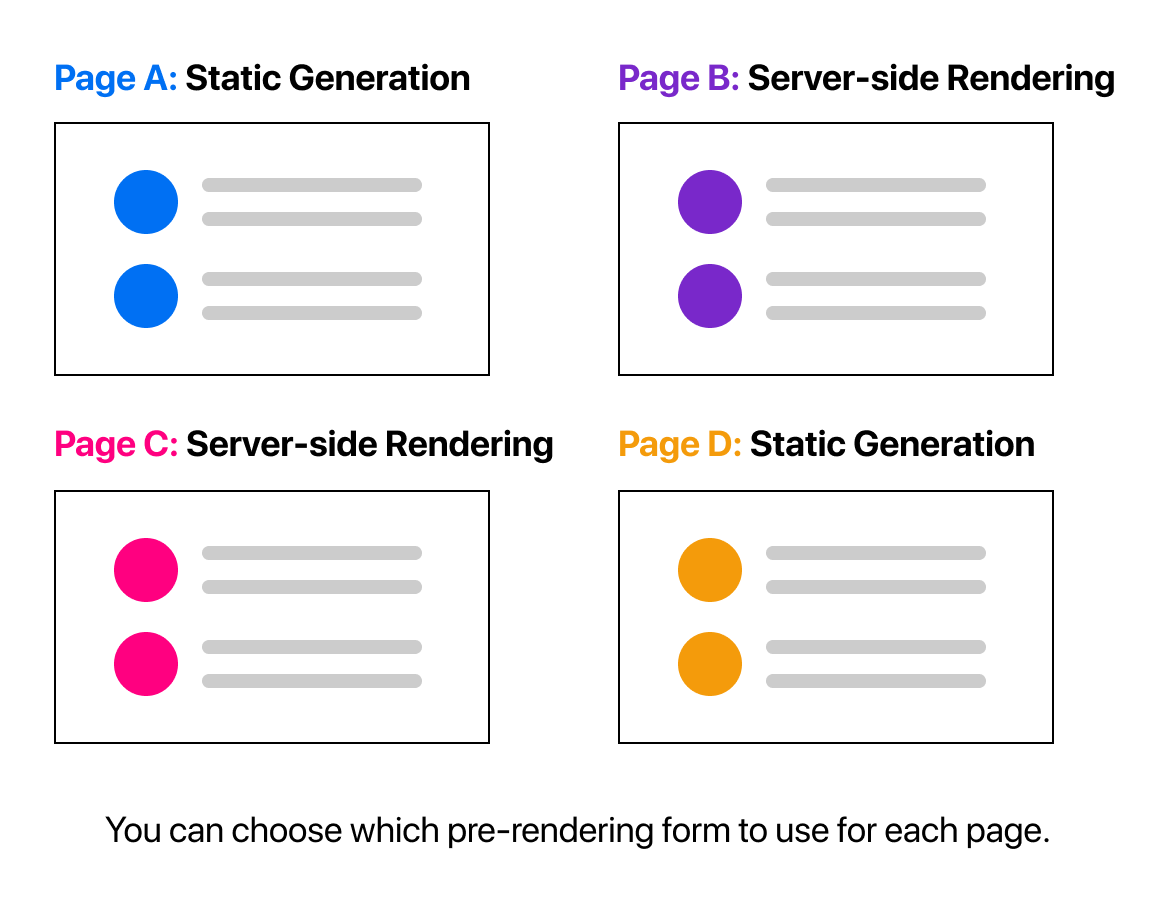
Importantly, Next.js lets you choose which pre-rendering form to use for each page. You can create a "hybrid" Next.js app by using Static Generation for most pages and using Server-side Rendering for others.
When to Use Static Generation v.s. Server-side Rendering We recommend using Static Generation (with and without data) whenever possible because your page can be built once and served by CDN, which makes it much faster than having a server render the page on every request.
You can use Static Generation for many types of pages, including:
- Marketing pages
- Blog posts
- E-commerce product listings
- Help and documentation
You should ask yourself: "Can I pre-render this page ahead of a user's request?" If the answer is yes, then you should choose Static Generation.
On the other hand, Static Generation is not a good idea if you cannot pre-render a page ahead of a user's request. Maybe your page shows frequently updated data, and the page content changes on every request.
In that case, you can use Server-side Rendering. It will be slower, but the pre-rendered page will always be up-to-date. Or you can skip pre-rendering and use client-side JavaScript to populate frequently updated data.
Rich Data Fetching Capabilities
Static Generation with and without Data
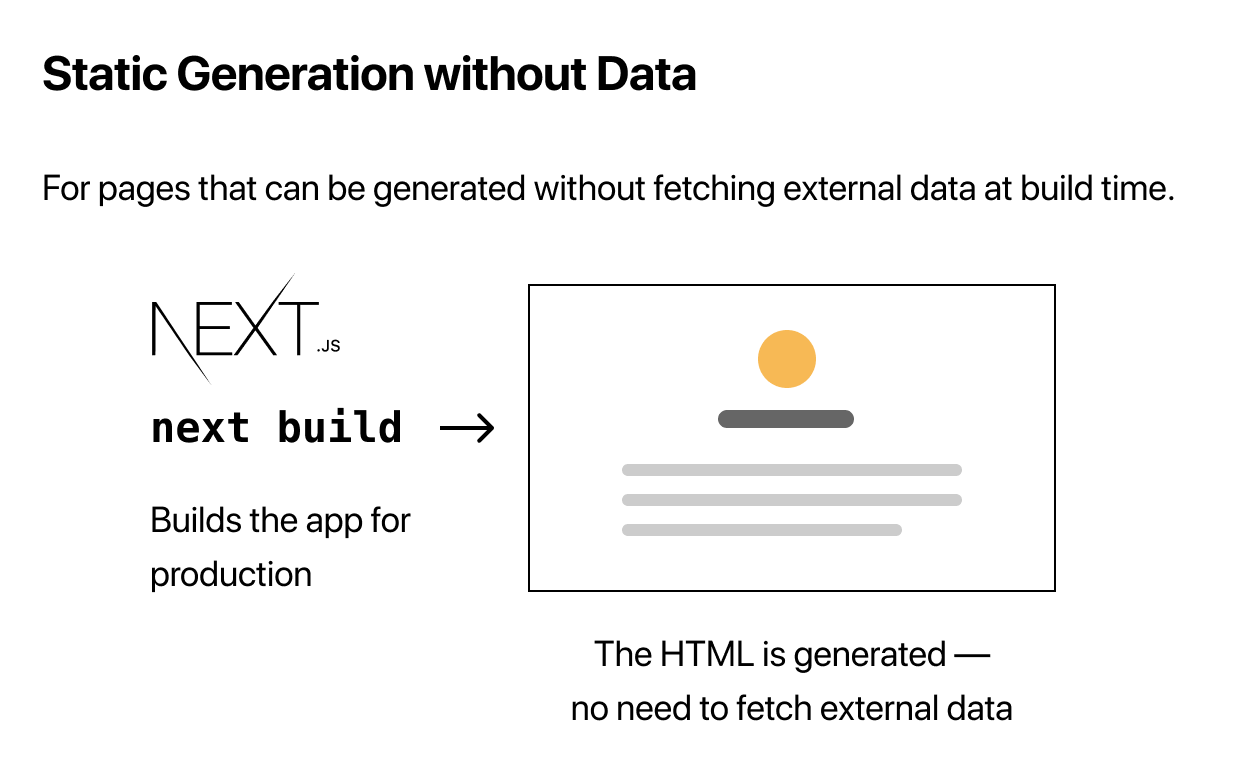
So far, all the pages we’ve created do not require fetching external data. Those pages will automatically be statically generated when the app is built for production.
Static Generation with and with Data
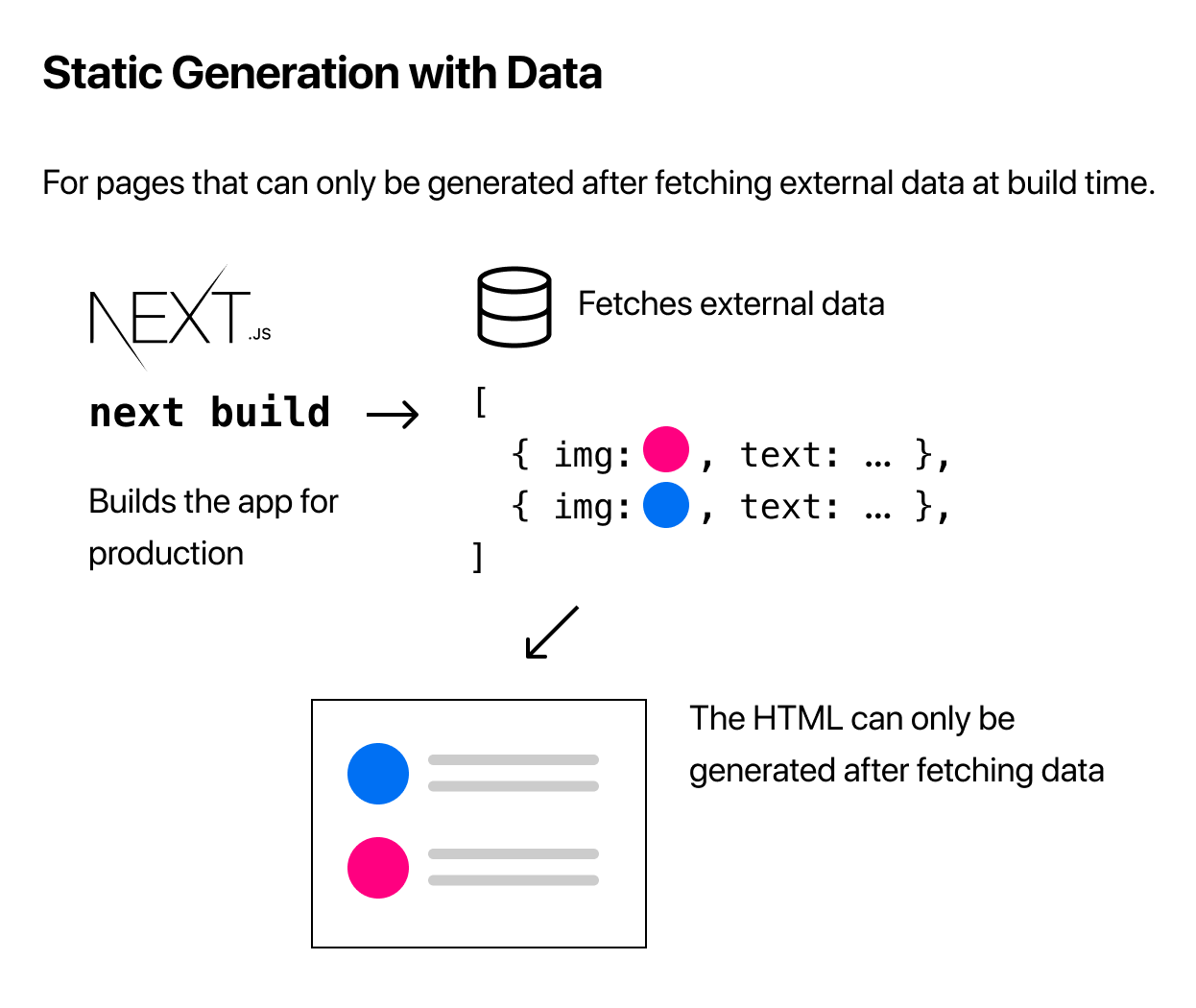
However, for some pages, you might not be able to render the HTML without first fetching some external data. Maybe you need to access the file system, fetch external API, or query your database at build time. Next.js supports this case — Static Generation with data — out of the box.
Static Generation with Data using getStaticProps How does it work? Well, in Next.js, when you export a page component, you can also export an async function called getStaticProps. If you do this, then:
- getStaticProps runs at build time in production, and…
- Inside the function, you can fetch external data and send it as props to the page
1export default function Home(props) { ... } 2 3export async function getStaticProps() { 4 // Get external data from the file system, API, DB, etc. 5 const data = ... 6 7 // The value of the `props` key will be 8 // passed to the `Home` component 9 return { 10 props: ... 11 } 12} 13
Information Sourced from: NextJS Docs